Events¶
Events are asynchronous messaging system based on publish subscribe messaging pattern. Publisher will publish a message and from no or all the subscribed consumers will receive the message. Events can work with rpc or http entrypoint for our example we are using rpc.
Source Code¶
We will create two service classes, first class would emit the event and second would receive it. Emitter class code will look like below
1 2 3 4 5 6 7 8 9 10 11 12 13 | # emmiter.py
from nameko import rpc, events
class EmitterService(object):
name = 'emitter_service'
event_name = 'some_event'
dispatch = events.EventDispatcher()
@rpc.rpc
def emit(self):
self.dispatch(self.event_name, 'payload')
|
Let’s define event handler class.
1 2 3 4 5 6 7 8 | # handler.py
class HandlerService(object):
name = 'handler_service'
@events.event_handler('emitter_service', 'some_event')
def handler(self, payload):
print('%s : %s' % (self.name, payload))
|
Setup¶
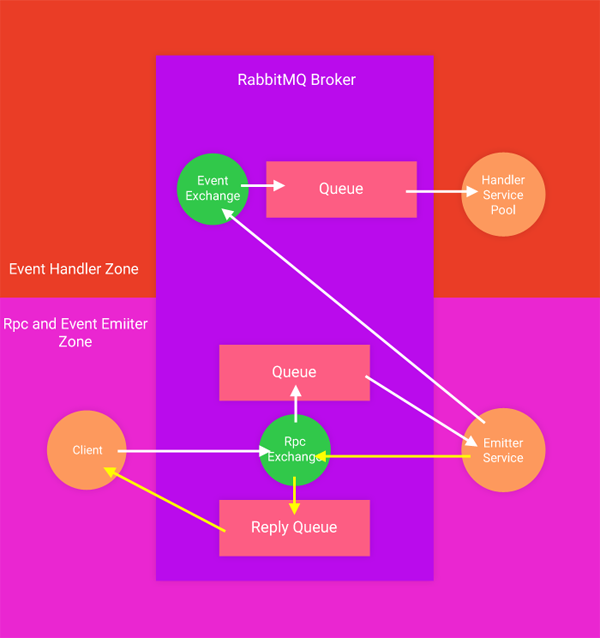
First, we will look at event handling part.
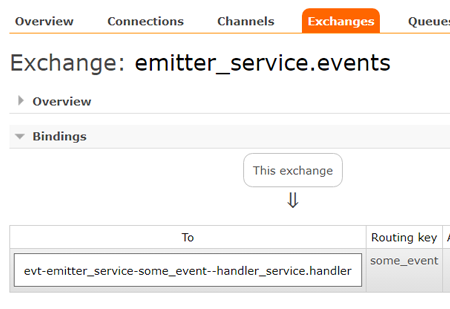
- starting
emitter_service
service will declare an exchangeemitter_service.events
(<producer name.event>). - running the
handler-service
will declare a queueevt-emitter_service-some_event--handler_service.handler
(evt-<producer name>-<event name>–<handler service>.<handler method>). - This handler queue will gets bound to
emitter_service.event
exchange with a routing key assome_event
(event name).
Next we will see how event_service
and rpc is setup.
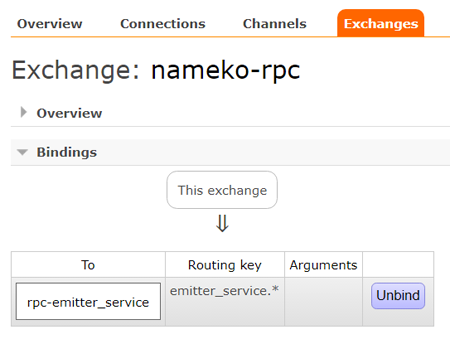
- This is the standard setup with
nameko-rpc
exchange bind torpc-event_service
queue. - Client declares its reply queue with
nameko-rpc
exchange.
Working¶
In nameko shell
we call the following command.
n.rpc.emitter_service.emit()
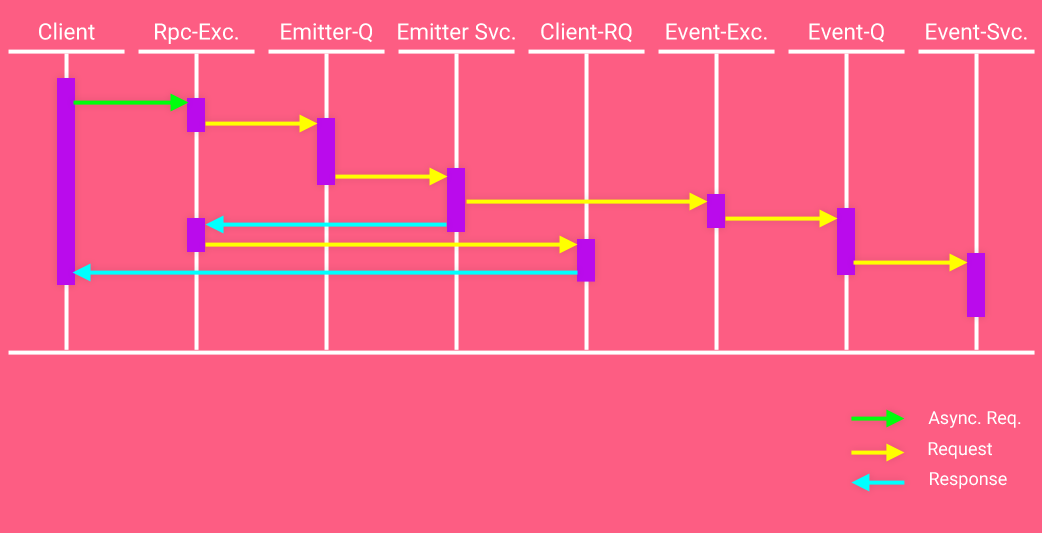
- Client makes an asynchronous call to rpc exchange
nameko-rpc
. - Exchange routes the message to
rpc-emitter_service
queue. - Queue then, routes it to the
emit
service method ofemitter_service
. emit
service method dispatches a new message toemitter_service.events
with routing keysome_event
(event name).- Event exchange then routes it to the event handler queue
evt-emitter_service-some_event--handler_service.handler
. - Queue then sends the message to the handler method
handler_service.handler
for processing.